コード
コードは前回のを少し変えてこうなります。
using SlimDX.Direct3D11;
using SlimDX.DXGI;
class Program
{
static void Main()
{
using (Game game = new MyGame())
{
game.Run();
}
}
}
class MyGame : Game
{
protected override void Draw()
{
GraphicsDevice.ImmediateContext.ClearRenderTargetView(
RenderTarget,
new SlimDX.Color4(1, 0, 0, 1)
);
SwapChain.Present(0, PresentFlags.None);
}
}
class Game : System.Windows.Forms.Form
{
public SlimDX.Direct3D11.Device GraphicsDevice;
public SwapChain SwapChain;
public RenderTargetView RenderTarget;
public void Run()
{
initDevice();
SlimDX.Windows.MessagePump.Run(this, Draw);
disposeDevice();
}
private void initDevice()
{
MyDirectXHelper.CreateDeviceAndSwapChain(
this, out GraphicsDevice, out SwapChain
);
initRenderTarget();
}
private void initRenderTarget()
{
using (Texture2D backBuffer
= SlimDX.Direct3D11.Resource.FromSwapChain<Texture2D>(SwapChain, 0)
)
{
RenderTarget = new RenderTargetView(GraphicsDevice, backBuffer);
}
}
private void disposeDevice()
{
RenderTarget.Dispose();
GraphicsDevice.Dispose();
SwapChain.Dispose();
}
protected virtual void Draw() { }
}
class MyDirectXHelper
{
public static void CreateDeviceAndSwapChain(
System.Windows.Forms.Form form,
out SlimDX.Direct3D11.Device device,
out SlimDX.DXGI.SwapChain swapChain
)
{
SlimDX.Direct3D11.Device.CreateWithSwapChain(
DriverType.Hardware,
DeviceCreationFlags.None,
new SwapChainDescription
{
BufferCount = 1,
OutputHandle = form.Handle,
IsWindowed = true,
SampleDescription = new SampleDescription
{
Count = 1,
Quality = 0
},
ModeDescription = new ModeDescription
{
Width = form.ClientSize.Width,
Height = form.ClientSize.Height,
RefreshRate = new SlimDX.Rational(60, 1),
Format = Format.R8G8B8A8_UNorm
},
Usage = Usage.RenderTargetOutput
},
out device,
out swapChain
);
}
}
まず、デバイスとスワップチェーンを作成します。 そしてスワップチェーン→リソース→レンダーターゲットという流れでオブジェクトを作成し、 レンダーターゲットを青でクリアします。 そして仕上げとしてスワップチェーンでプレゼントします。 そうするとウィンドウに青が反映されます。 結果はこうなります。
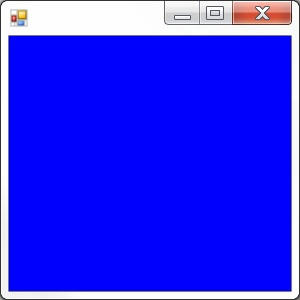